#include <dev_work>
/* Info:
* This page contains a selection of projects I've worked on. Currently these consist of both personal
* and academic works in various languages, with an obvious theme of graphics, physics and ML!
*/
Accelerating Fluid Simulations Using Deep Learning– MSc Dissertation Project
Languages: C++, CUDA, Python, MATLAB, GLSL Key Libraries: PyTorch, LibTorch, OpenGL
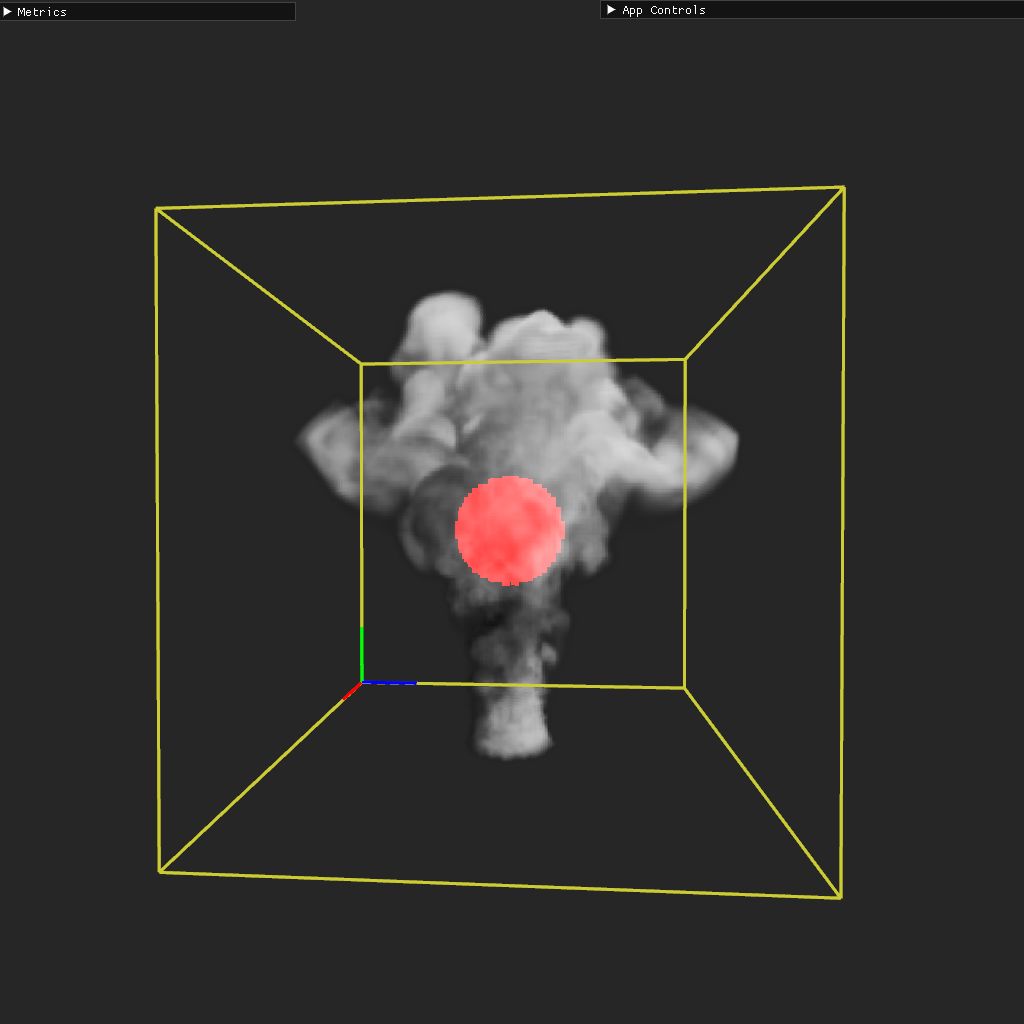
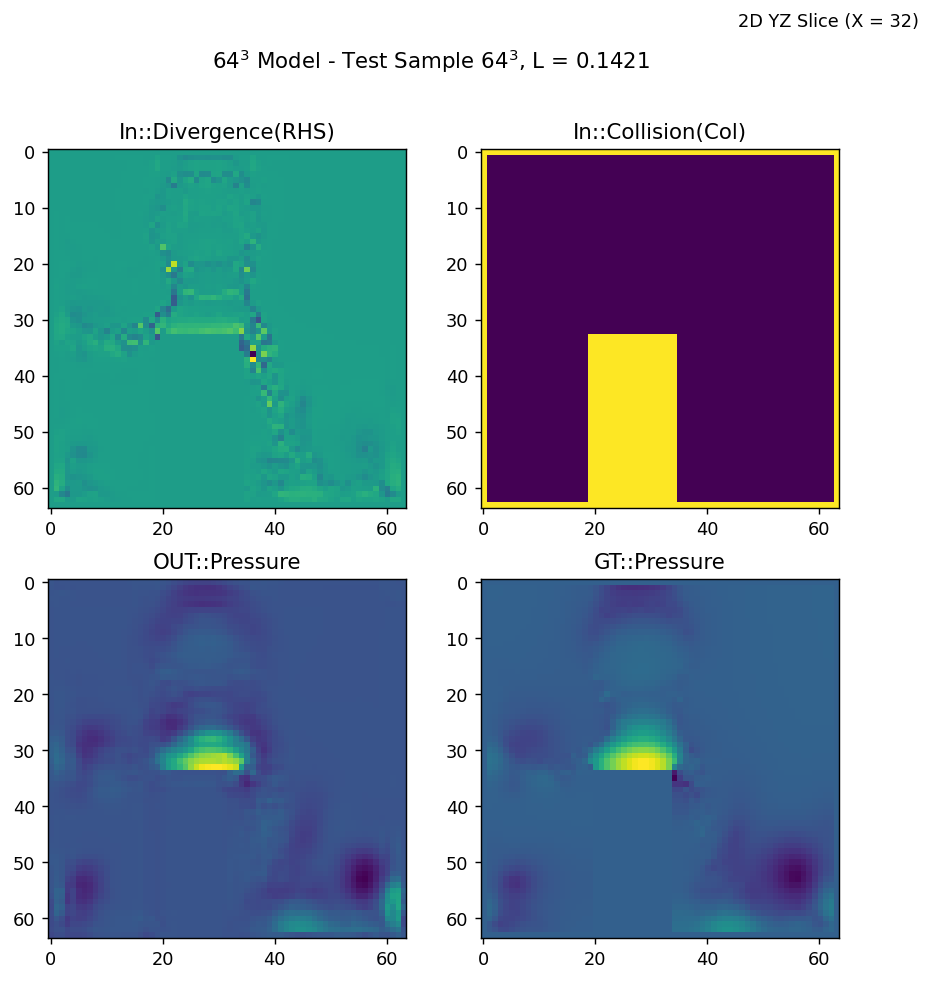
My MSc dissertation at the University of Leeds in late 2022, involved a self proposed, multi-stage project aiming to accelerate real-time fluid simulation using deep learning.
This work focused specifically on the acceleration of the pressure solve stage of ‘The Projection Method’ which involves calculating the unknown pressure field, given the current intermediate velocity field. This pressure field is derived from a Poisson equation, which typically requires running an expensive linear solver to numerically compute it. The convergence and thus residual of this solver is vital to produce an accurate pressure field, which projects the fluid velocity field onto its divergence free subspace to ensure incompressibility is maintained and thus realistic visual results in the flow are present.
My project takes inspiration from and cites [Tompson et al. 2017] with the aim to approximate this pressure field using a fully convolutional neural network, replacing the linear solver, with a fixed cost inference step that can utilise modern GPU architectures. The aim is that one day, this could be used to allow for high quality, high performance interactive fluid simulations within games and XR experiences, where performance and thermal constraints of hardware was previously bounded.
The project was a large engineering undertaking and involved several sub-projects:
- Fluid Simulation Framework – I wrote a custom simulation framework in C++ / CUDA from scratch, based on solving a reduced set of the Incompressible Naiver Stokes equations in real-time. This was used both to generate training data and perform the rest of the simulation operations at runtime as a small and modular library. This was optimised to ensure good usage of GPU resources, avoiding costly host-device transfers.
- Training Data Generation – A client application that calls the base fluid simulation framework library, to generate many permutations of the parameter space, generating thousands of simulations to form the training dataset. This data was used for training the neural network.
- Neural Network Training – The fully convolutional network architecture was built and trained within PyTorch using Python. Both quantitative and qualitive testing was used to validate and examine the resulting models, adjust hyperparameters and make architectural and training logic changes.
- Tech Demo Application – The trained model was utilised in a small program to test in an applied setting, where a gaseous smoke simulation could be interacted with by a user controller input. My fluid framework was utilised for simulation and the trained pressure FCN model was used for inference by exposing it to this C++ application through LibTorch (PyTorch’s C++ frontend). This was compared with common numerical linear solvers such as Red-Black Gauss-Seidel. Finally a basic volumetric renderer was written using OpenGL utilising ray marching to visualise the resulting fluid data.
This project was expanded to have nicer visualisation and rendering, along with initial support for a combustion model.
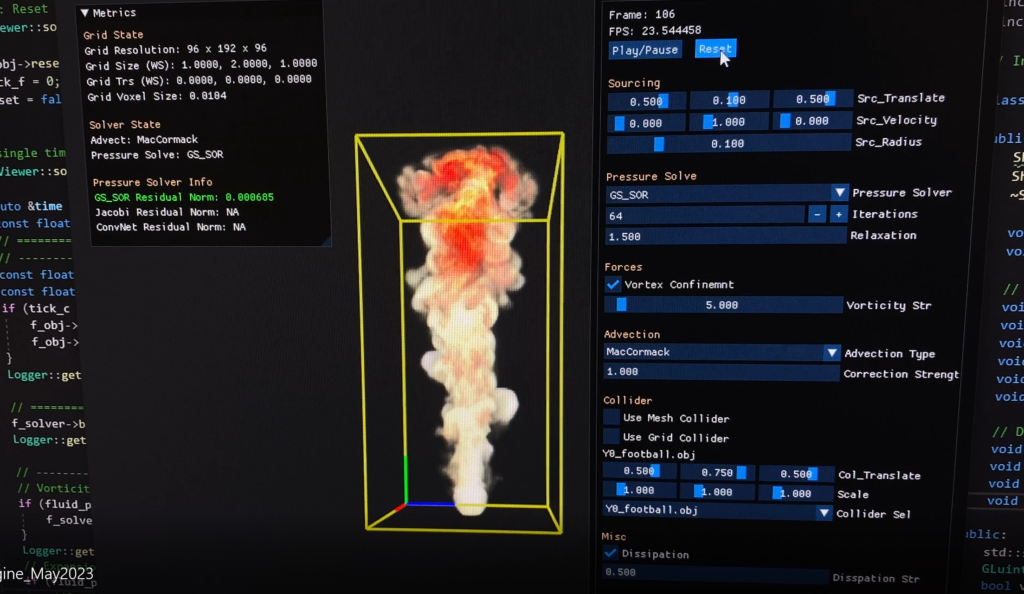
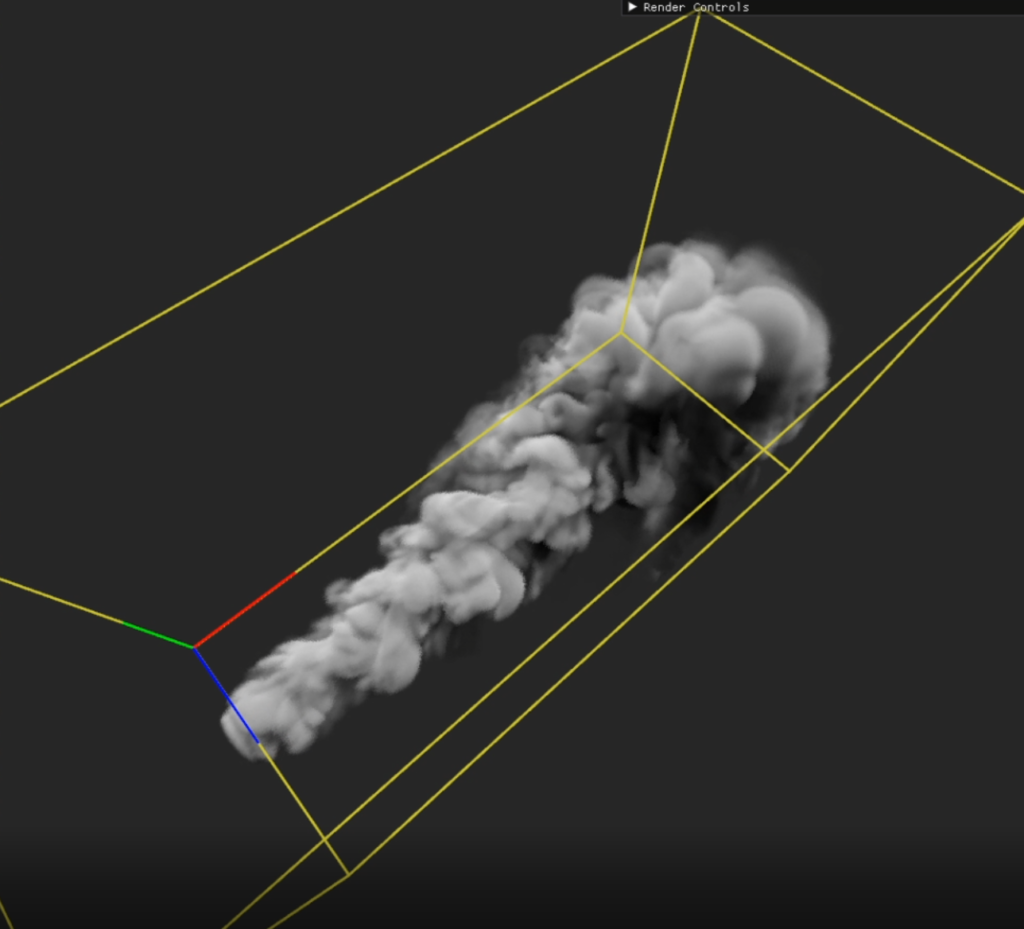
I’m pleased to announce that this dissertation resulted in a distinction grade. Due to the short time frame of UK MSc projects, I did revert to using a purely supervised learning approach and thus the work is not novel, however I am working on an internal fork that advances upon both my work and the SOTA I plan to share in the future.
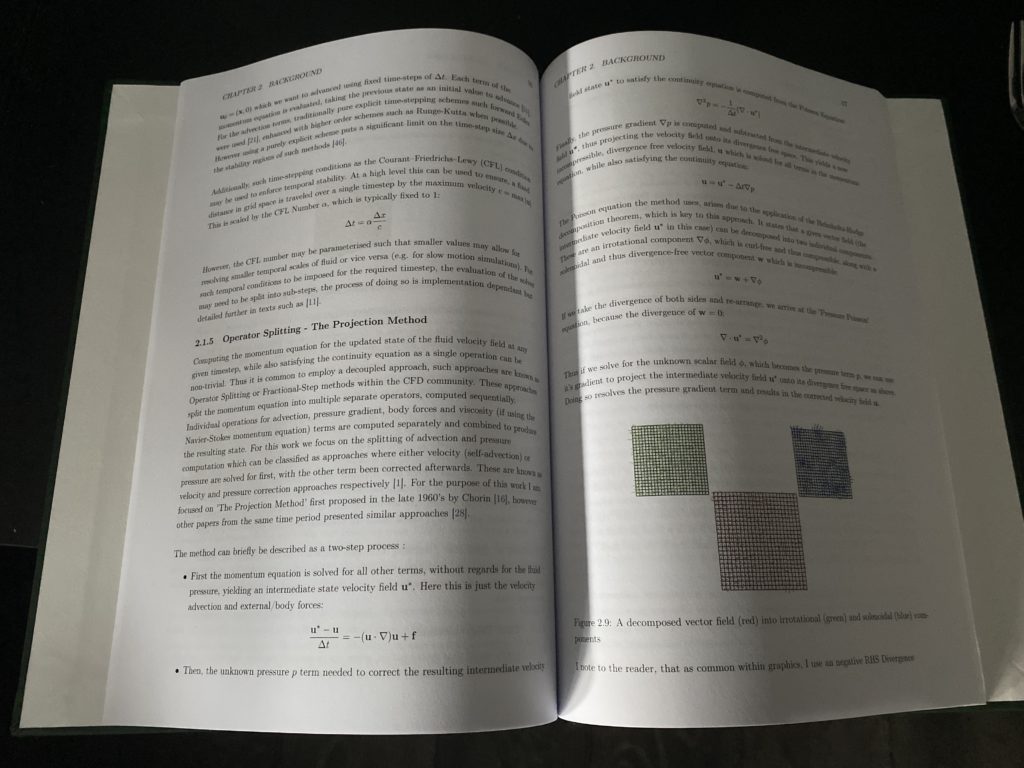
UE4 Cloth Simulation Plugin – A Cloth Solver using Position Based Dynamics with Verlet Integration.
Languages : C++, HLSL Libraries: UE4 C++ API.
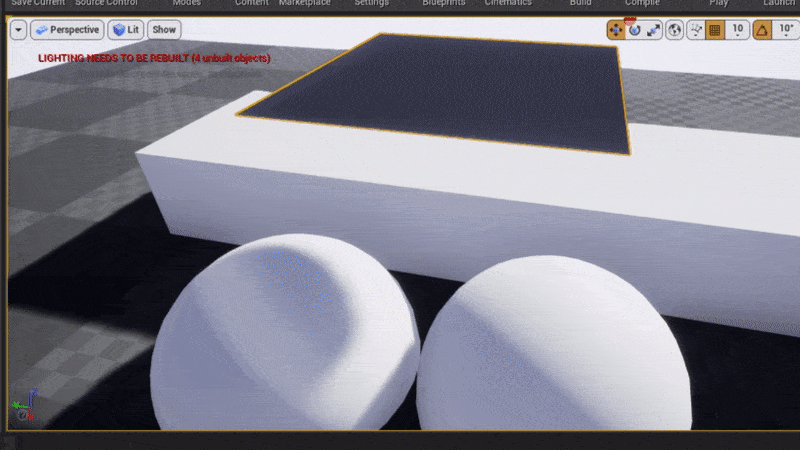
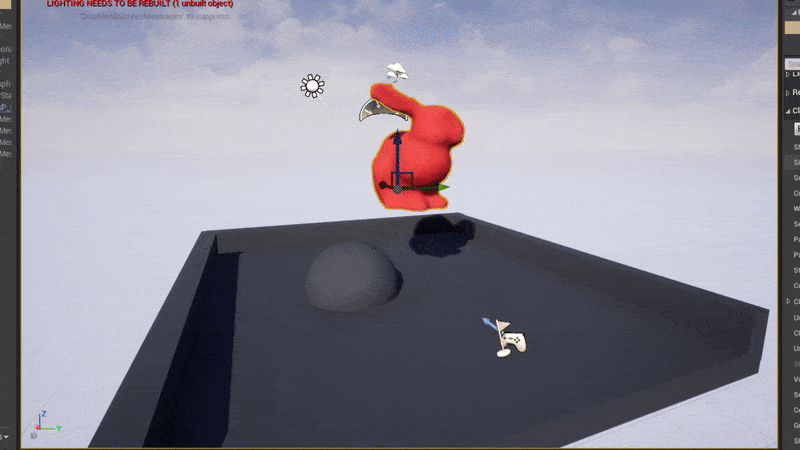
This project started out as a weekend project to write a small cloth solver plugin for Unreal Engine utilising Position Based Dynamics (PBD) that could be used to simulate both in-game and offline cloth simulations for purposes like set dressing within the editor, along with runtime user interaction. The input mesh takes a standard triangular mesh, constraints are constructed automatically using the topology. The resulting simulation can be paused and converted back to a static mesh.
The plugin also takes advantage of the Procedural Mesh Component within Unreal Engine’s API, to allow efficient updates and rendering of mesh data. I focused some time optimising it and implementing various features including self collisions (with spatial acceleration) and volume pressure force. The integration relies on the velocity-less Verlet scheme, which is second order in time and avoids the need to store velocities per particle. This however comes with some downsides, so traditional, first order, Semi-Implicit Euler is also provided.
Additionally prior to starting university I worked on accelerating the constraint solve using GPU via compute shaders (Written in HLSL, cross compiled to multiple platforms), while this has not been pushed yet some results can be seen above. I plan to clean this project up, move to Unreal Engine 5 and document some of the coding process in the near future.
Stable Fluids GL – A CPU based fluid simulation framework with OpenGL based Volume Rendering.
Languages: C++, GLSL Key Libraries: OpenGL, GLFW, OpenMP
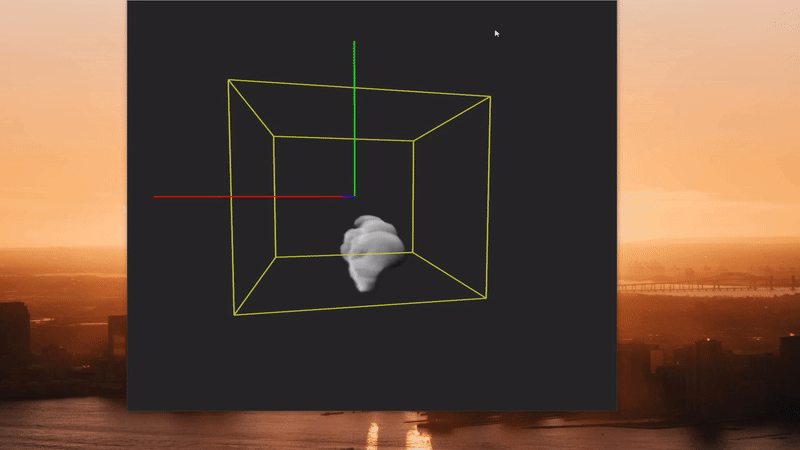
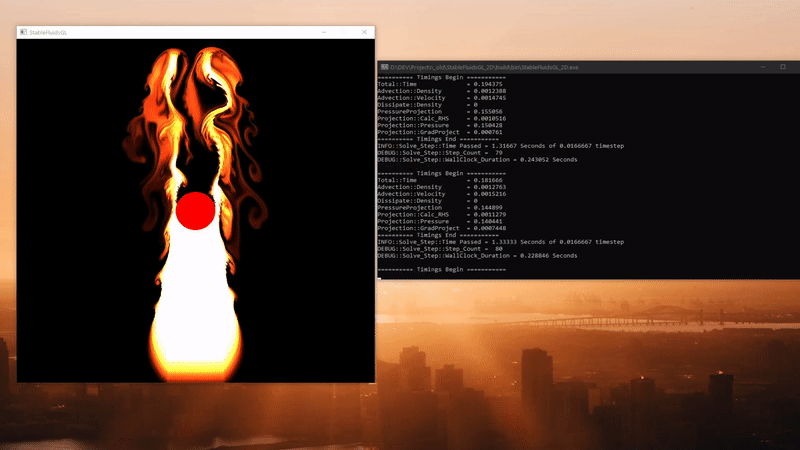
This project is based on the work of Jos Stam and his seminal ‘Stable Fluids’ paper. This project is where my interest with linear solvers solving the ‘Pression Poisson’ equation began! I use this framework as reference for any fluid related project’s I work on as I find it handy to have a CPU based framework I can use for ground truthing when implementing more higher performance code.
Some key features :
- Semi Lagrangian Advection
- MacCormack Advection
- Vorticity Confinement
- Pressure/Poisson Equation Linear Solvers – Jacobi, Gauss-Seidel, SOR
- Volumetric Rendering – Ray Marching (OpenGL)
- Caching to a compact binary format.
I also wrote some specific SIMD implementations using SSE and AVX for accelerating commonly used functions like lerp, clamp, fit value range and so forth, to take advantage of vectorisation on x86/x64.
COMP5530M Game Engine – Rendering/Material System – My contribution to the COMP5530M group project at University of Leeds, 2022.
Languages: C/C++, GLSL Key Libraries: OpenGL, GLFW, Dearimgui
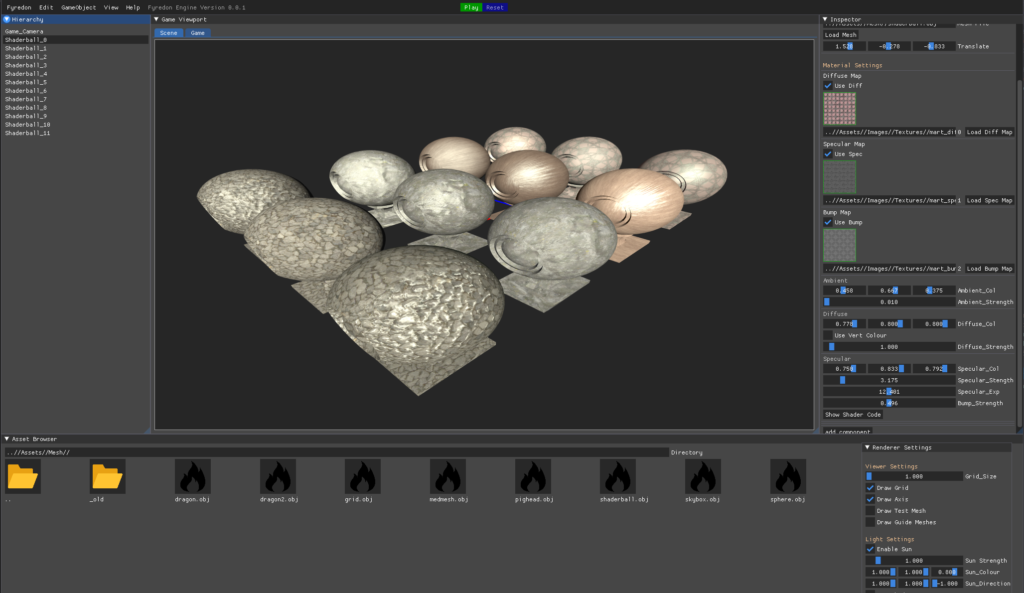
I wrote the rendering and material system, I also led the the team for the first half of the project; this involved setting up an Agile like methodology for approaching the software engineering process of the project. I also implemented the base application and windowing. The rendering subsystem implemented an abstracted interface to the underlying graphics API which was OpenGL 4.5. Due to time sake the material system is non-PBR, but still allows for creating variations in materials that look physically plausible.
- Abstraction of Graphics API Backend – Implements high level abstractions for common primitives and constructs defined by modern graphics APIs (Textures, Framebuffers, Meshes, Lights). The engine specifically targets OpenGL 4.5, but other API back-ends could be added by implementing these abstractions.
- Auto Mesh Indexing System – Allows meshes with multiple vertex attributes to be automatically indexed when loaded into the engine, only keeping unique vertices, this was implemented using a hashing based approach and allowed large memory savings when dealing with meshes with lots of shared attributes, such as the terrain subsystem.
- Material System – Users can load texture maps, define parameters to create various perturbations of material properties, this is accessible by a GUI panel interfacing the engine editor application with editable parameters.
- Hot-Reloading of Shaders – Re-compile shaders using static code paths to prevent dynamic branching on the GPU in response to material parameters changing.
The project is hosted on GitLab by a teammate.
COMP5823M Animation & Simulation Projects
Languages: C/C++, GLSL Key Libraries: OpenGL, GLFW, Dearimgui
For coursework during COMP5823M at University of Leeds, I produced 3 projects related to ‘Animation & Simulation’. Given my prior experience, these are far from my most complex work, but they turned out as quite nice standalone applications given the short turnaround time.
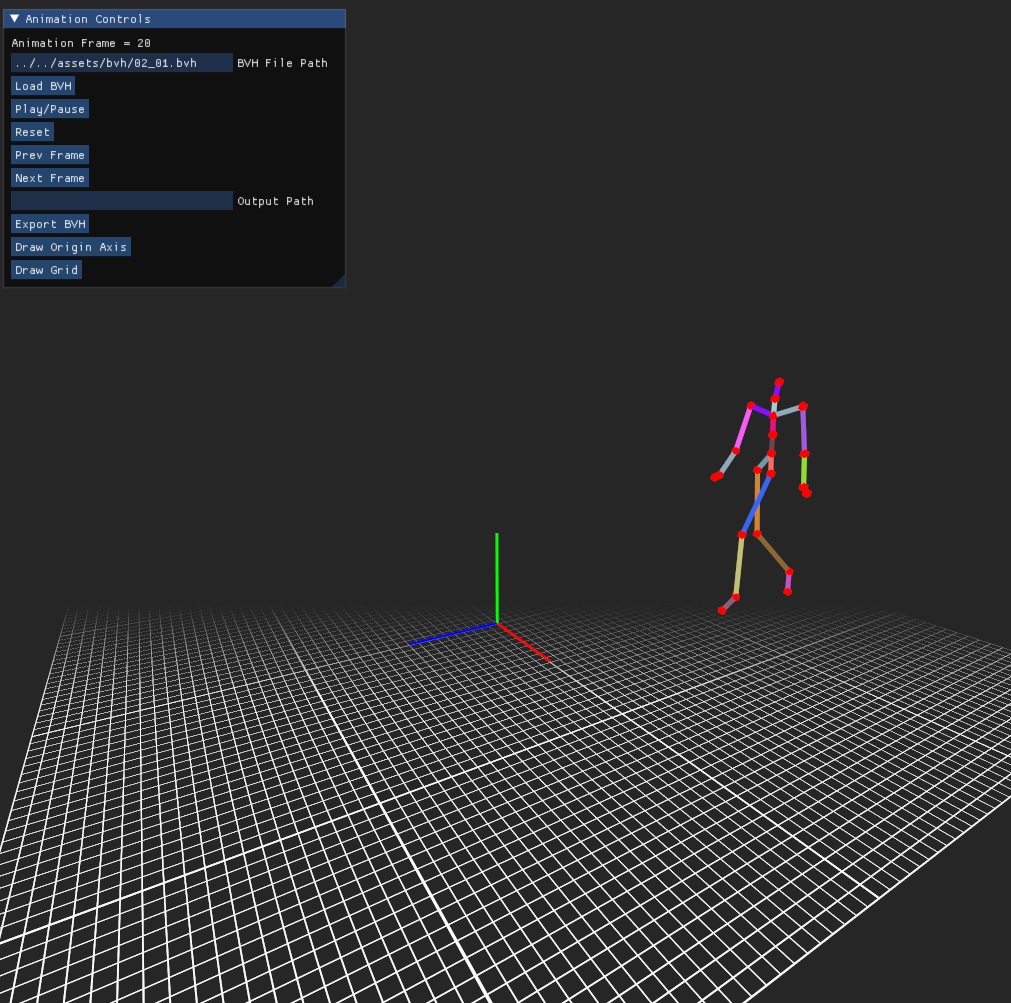
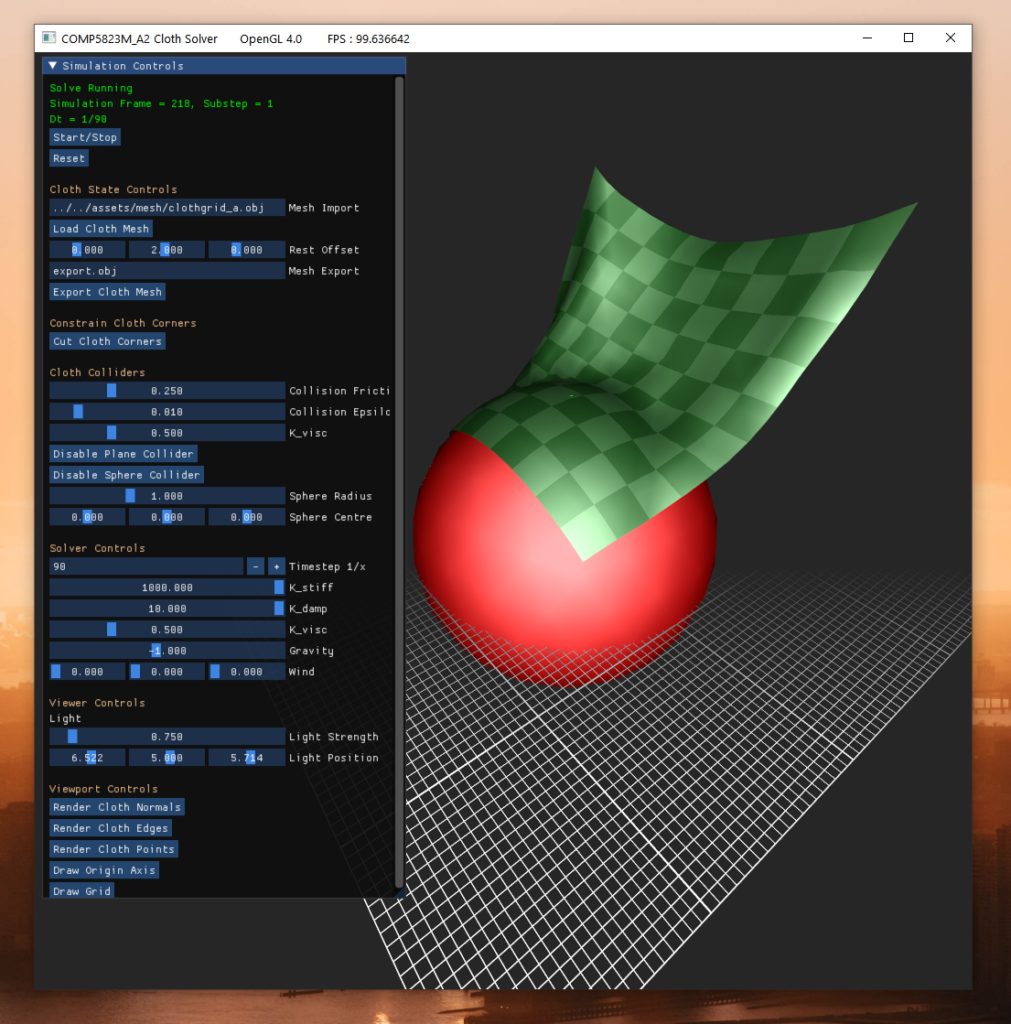
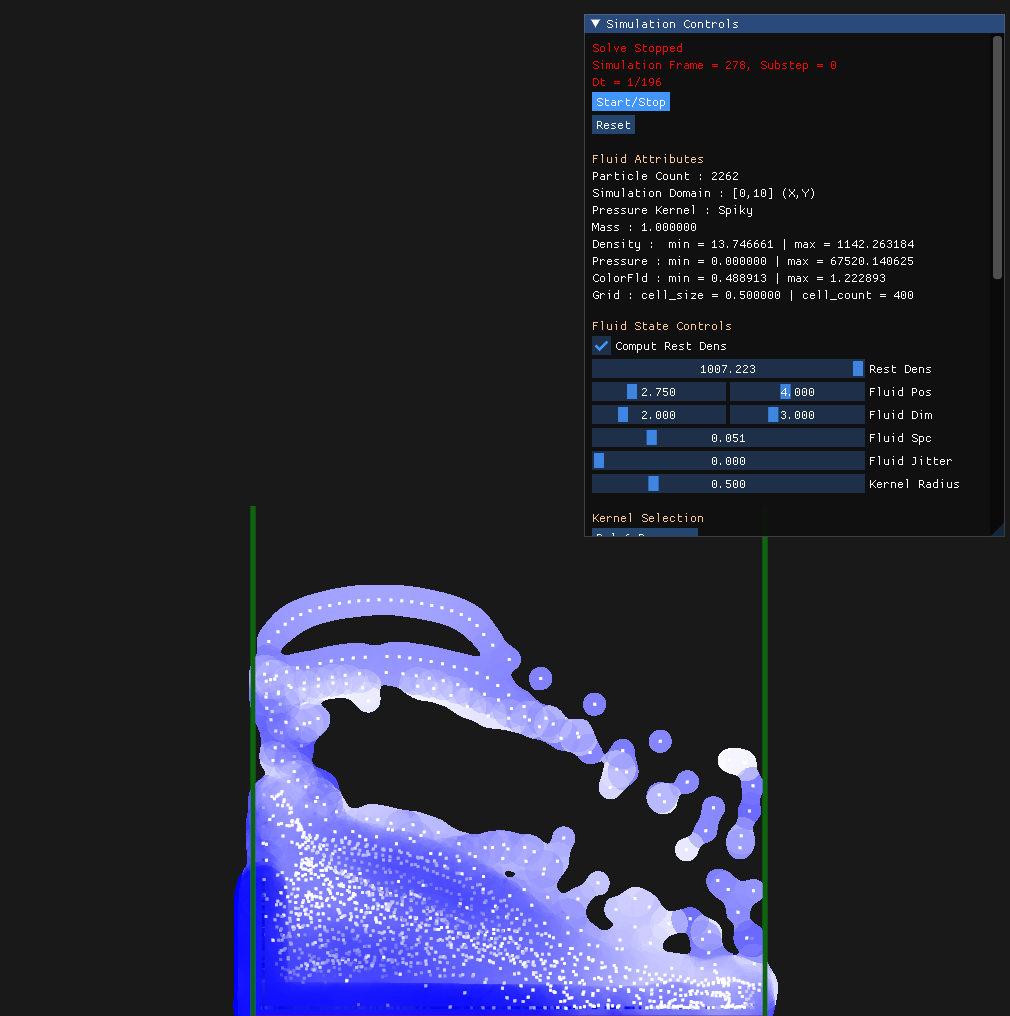
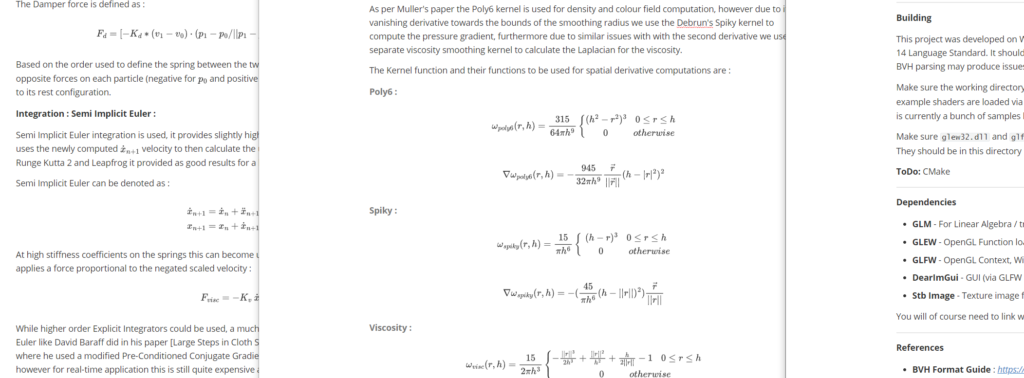